Copy a PocketQuery table as CSV
Sometimes it is useful to “take a snapshot” of a PocketQuery macro and persist it somewhere for later use.
The macro does not come with a default option that allows you to do this, but it can easily be achieved with a simple PocketQuery Template.
Using this Template, you can generate a button that allows you to copy the whole PocketQuery table as CSV with a single click.
<script>
AJS.toInit(function() {
// Copies text into the user's clipboard
function copyToClipboard(text) {
var temp = jQuery("<textarea />").appendTo("body").val(text).select();
document.execCommand("copy");
temp.remove();
jQuery("#pq-copy-as-csv-msg").show();
}
// Transform PQ result data into CSV
function generateCsv() {
var headers = PocketQuery.queryColumns().join(',');
var rows = PocketQuery.queryArray().map(function(row) {
return Object.keys(row).map(function(column) {
return row[column];
}).join(',');
}).join('\n');
return headers + '\n' + rows;
}
// Bind functionality to our button
jQuery("#pq-copy-as-csv").on("click", function() {
var csv = generateCsv();
copyToClipboard(csv);
});
});
</script>
<button id="pq-copy-as-csv" class="aui-button aui-button-primary">Copy table as CSV</button>
<span id="pq-copy-as-csv-msg" style="display: none;">Copied into clipboard!</span>
## Render the PocketQuery default Template (table)
$PocketQuery.template("default")
This is what the result will look like:
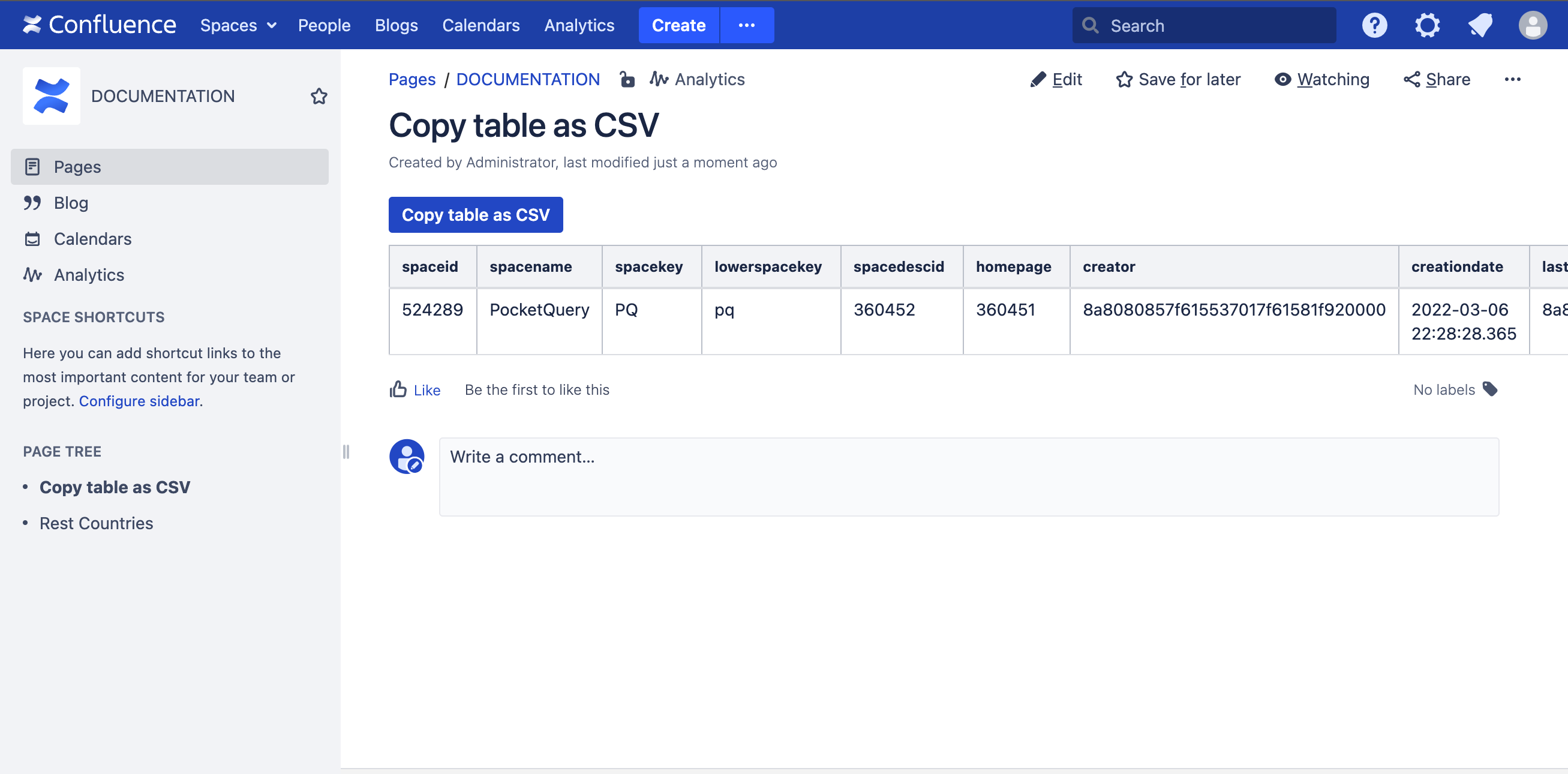