Load all data from a paginated Rest API
It sometimes happens that Rest APIs are paginated. In this example, we want to load all data from an API that returns 10 items per page and to load the following items, we need to add the query parameter page to load it (i.e. /items?page=2).
We need to create two queries and a template for query 1:
Query 1: Load first page from API
Query 2: Load pages from API with page parameter
Template: Load data recursively from query 2
Query 1: Load first page from API
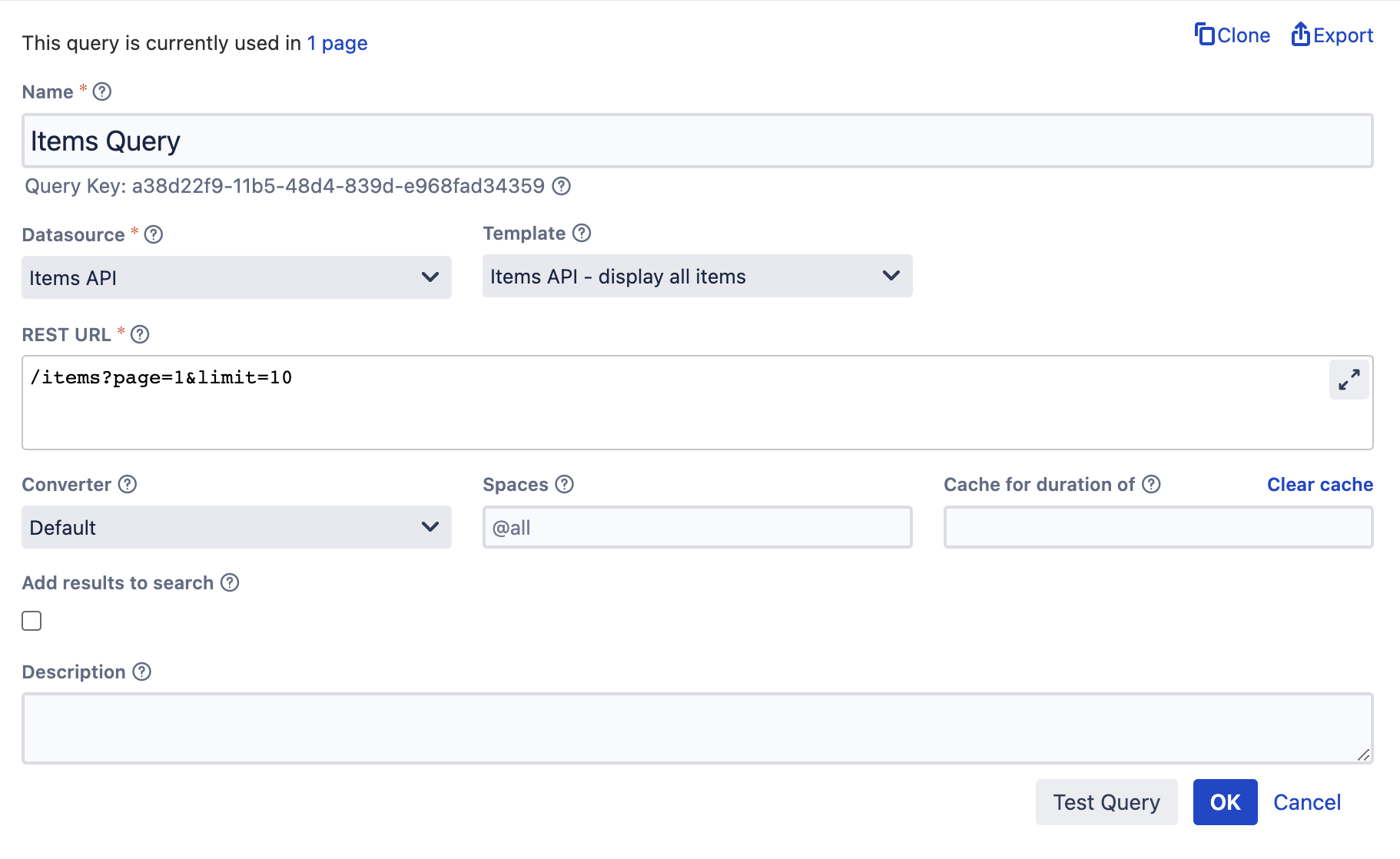
Query 2: Load pages from API with page parameter
The second query will be executed inside the template and contains a parameter to load the consecutive pages.
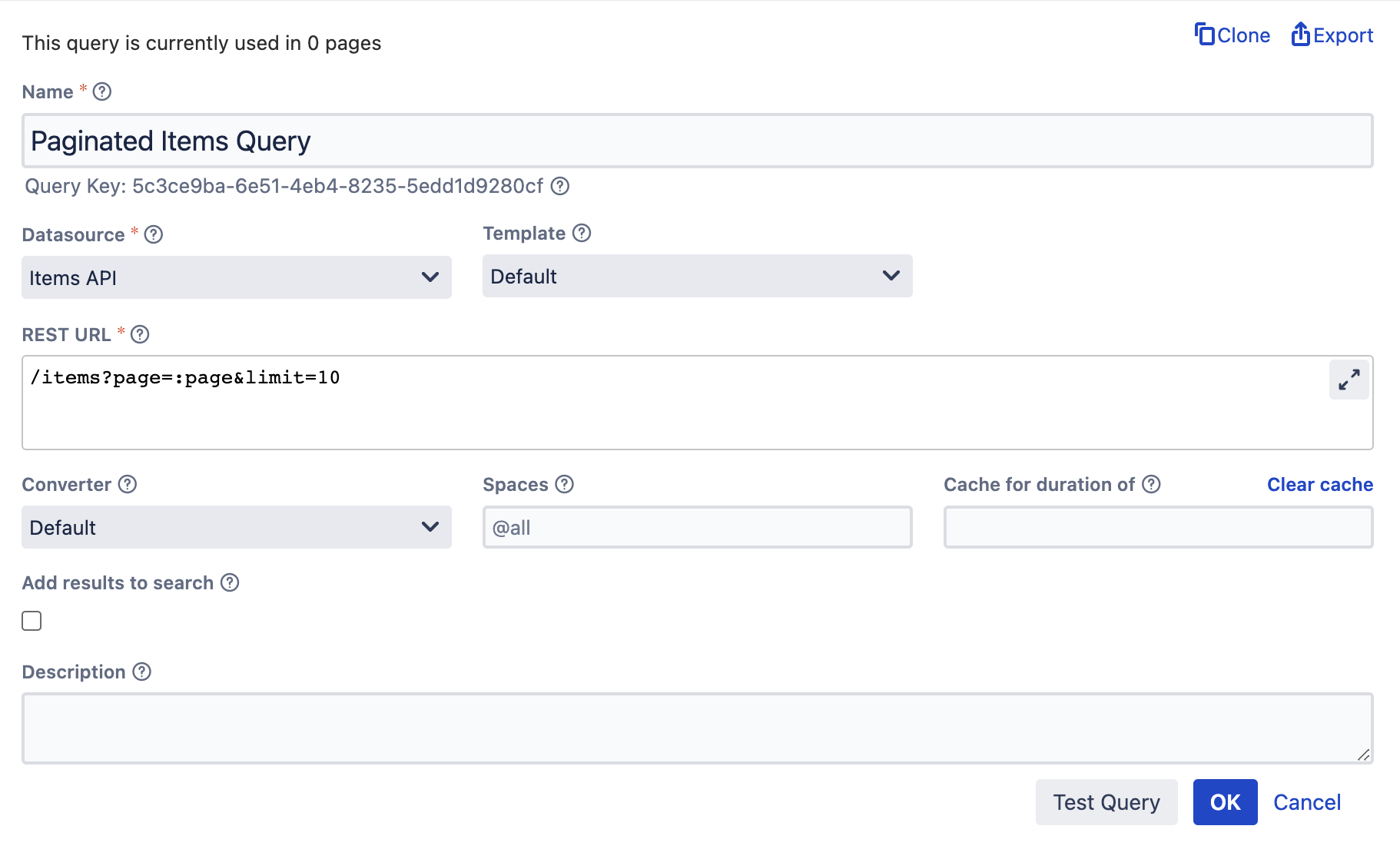
Template: Load data recursively from query 2
The key-part in this solution is the template. Here, we will execute the second query recursively until there are less than 10 items returned:
#macro(fetchResultsRecursively $page)
## call second query. the first param is the query key, which can be found under the query name
#set($pageResult = $PocketQuery.executeQuery("5c3ce9ba-6e51-4eb4-8235-5edd1d9280cf", {
"page": $page
}))
## add the results from the current page to the results from the initial query
#set($ok = $result.addAll($pageResult))
#if($pageResult.size() == 10)
## increment the page and load the next results
#set($page = $page + 1)
#fetchResultsRecursively($page)
#end
#end
## start recursion with the second page
#fetchResultsRecursively(2)
<table class="aui confluenceTable">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Status</th>
</tr>
</thead>
<tbody>
#foreach ($row in $result)
#if ($row.status == 1)
<tr>
<td>$row.id</td>
<td>$row.name</td>
<td>$row.status</td>
</tr>
#end
#end
</tbody>
</table>