Converters
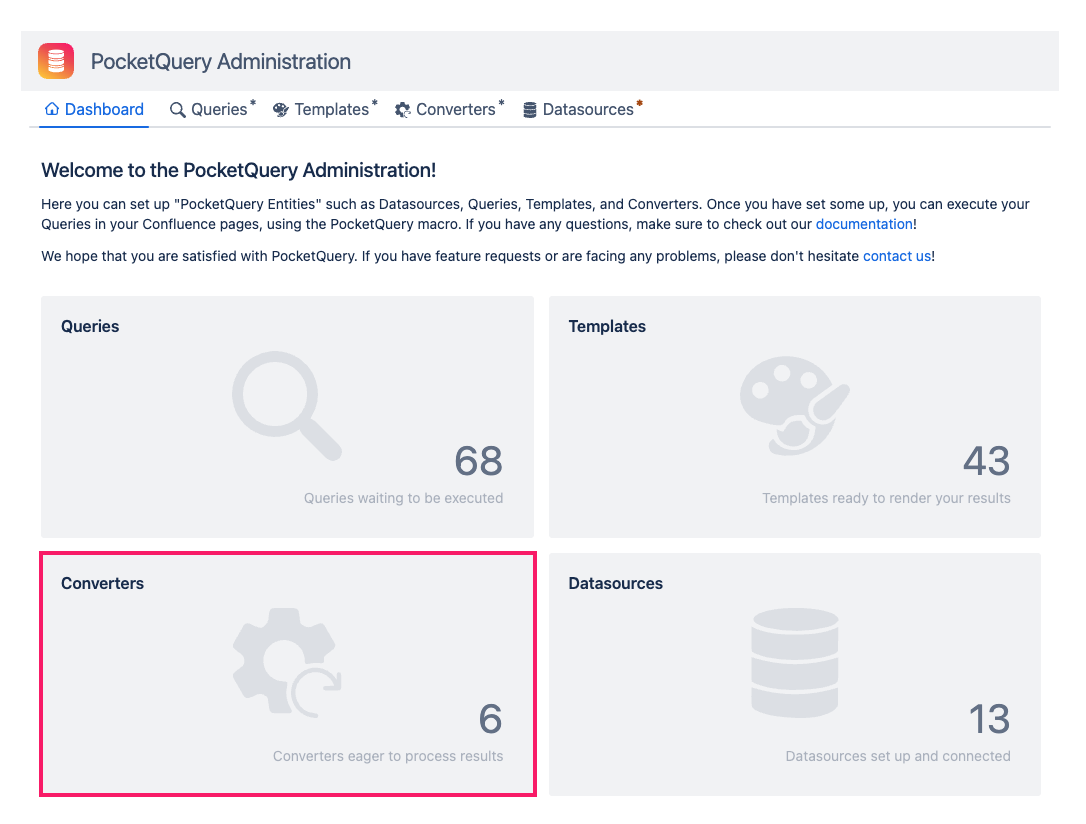
Converters are a type of PocketQuery Entity that defines how a Query result shall be processed before it’s rendered. They are JavaScript functions and receive the Query result as JSON.
If you have never used JavaScript before, you should make yourself familiar with the basics. We also recommend looking at the JavaScript API available in Converters and our Converter Tutorial.
Default Converter
If you don’t specify a Converter yourself, PocketQuery will route your result data through a default Converter. This converter has the following code:
function convert(json) {
const parsed = JSON.parse(json);
return parsed;
}
This code only converts the result to an object. It does not change the provided data structure in any way.
Example Converter
If you decide to implement an own Converter, you can find the “Example Converter” a handy starting point. The “Example Converter” is a simple piece of code inserted into your editor when you hit the “Insert Example Code” button.
function convert(json) {
const parsed = JSON.parse(json);
return parsed['issues'].map(issue => ({
'Issue Key': issue.key,
'Summary': issue.summary,
...
}));
}
Converter Metadata
Converters may optionally receive a second object as argument that will contain the following data:
queryName (String) | The name of the query currently executing this converter |
queryParameters (Object) | The parameters of the query currently executing this converter |
originalQueryParameters (Object) | Same as queryParameter but before any processing took place (e.g. wildcard replacements) |
Example:
function convert(json, metadata) {
return [{
"QueryName": metadata.queryName,
"QueryParameter Continent": metadata.queryParameters.Continent,
"QueryParameter MinPopulation": metadata.queryParameters.MinPopulation,
"Original QueryParameter Continent": metadata.originalQueryParameters.Continent,
"Original QueryParameter MinPopulation": metadata.originalQueryParameters.MinPopulation
}];
}
If you are interested in resource limits related to Converters, check out this article.